.NET is probably one of the more muddled and mismanaged brands in the history of Microsoft. If you go to microsoft.com it will tell you that ".NET is the Microsoft Web services strategy to connect information, people, systems, and devices through software," but this isn't what most people are thinking of when they say, ".NET." What is commonly referred to as .NET is programming with the .NET Framework. This is what I am going to cover in this article. If you are looking for marketing speak then please refer to www.microsoft.com/net.
The Runtime
At the heart of .NET is the Common Language Runtime, commonly referred to as the CLR. The CLR is made up of a number of different parts, which I will be covering here piece by piece (if you didn't want a technical article then you should've followed the marketing link).
Visual overview of the Common Language Infrastructure (CLI)
Language Independence
One of the most important facets of the .NET Framework is language independence. You can write .NET applications using any number of different programming languages. The most popular languages tend to be C# and VB.NET, but many other languages now have .NET versions including Python, COBOL, and more. You can see a list of many of the languages you can use with .NET over at dotnetpowered.com/languages.aspx.
Language independence is attained through the use of an intermediate language (IL). What this means is that instead of code being compiled in actual machine code (code that the CPU would run), it is instead compiled into a high-level generic language. This means that whatever language you write your code in, when you compile it with .NET it will become IL. Since all languages eventually get translated into the intermediate language, the runtime only has to worry about understanding and working with the intermediate language instead of the plethora of languages that you could actually use to write code.
Just-in-Time Compilation
If your mantra is, "Why do something now you can put off till tomorrow?" then you have something in common with the CLR. When you compile your code and it is translated to the intermediate language it is then simply stored in an assembly. When that assembly is used the CLR picks up that code and compiles it on-the-fly for the specific machine that is running the code. This means the runtime could compile the code differently based on what CPU or operating system the application is being run on. However, at this point the CLR doesn't compile everything in the assembly; it only compiles the individual method that is being invoked. This kind of on-the-fly compilation, referred to as jitting, only happens once per method call. The next time a method is called, no compilation occurs because the CLR has already compiled that code.
Memory Management
One of the constant assailants on productivity in unmanaged programming platforms is manually managing memory. Having to deal with memory management is also one of the largest sources for bugs and security holes in many applications. .NET removes the hassle of manually managing memory through the use of the aptly named garbage collector. Instead of the developer needing to remove objects from memory, the garbage collector looks at the current objects in memory and then decides which ones aren't needed anymore. For some developers this will be a tough pill to swallow; if you are used to managing memory then turning it over to an automated process can be somewhat troubling. This is when you have to take a step back, stop worrying, and embrace the runtime. There are bigger problems to solve (namely the business problems that are probably the real goal).
Alternative CLR Implementations
The .NET runtime is actually based on a standard developed by Microsoft called the CLI or Common Language Infrastructure, portions of which have been submitted to Ecma as an international standard. Because the CLR is based on an open standard, there have been a number of alternative CLR implementations, most notably Rotor and Mono. Rotor was a project from Microsoft Research, is a version of the CLR that will run on Mac OS, and is shared source. Mono is an independent open source implementation of the CLR that runs on various Linux distributions. While "Write once, run away" is not always realistic with .NET, there are some options available when it comes to other platforms. (Some code can be moved without issue, but most will require some tweaking, as different implementation includes different functionality.)
The Library
While the runtime is definitely the most important part of .NET, you can't do too much with it by itself. This is where the Base Class Library (or BCL) comes in. The BCL includes a lot of the plumbing of .NET, including the system types, IO, and functions for working with text. In addition to the BCL, there is the Framework Class Library (FCL). The FCL is an extended library that makes working with the .NET Framework practical and includes the following major pieces:
ADO.NET
Most current applications involve working with databases, normally more than one. ADO.NET is the data access component of the .NET Framework and includes built-in providers for SQL Server, ODBC, OLEDB, as well as Oracle.
Windows Forms
Windows forms are the .NET Framework method for building desktop-based applications. Windows Forms are simply a managed wrapper over the native Windows API, this means that you can write code for one version of Windows and it will run on other versions without issue all the way back to Windows 98 SE.
Windows forms applications do require the .NET Framework to run, which means that anyone who downloads your application, or any computer it is installed on, will need to also have the .NET Framework. The framework can be easily installed through Windows Update, and is completely free, but the download size can be troublesome for people with a slower connection.
ASP.NET
ASP.NET is the part of the .NET Framework dedicated to building web applications. Using ASP.NET you can build everything from a small starter website to enterprise-level web applications. ASP.NET allows you to write web applications without the need for a scripting language, everything can be written in your .NET language of choice.
Since ASP.NET applications are simply rendering HTML for the browser, there is no requirement for the .NET Framework on the client. Chances are you have used a number of sites that have been written in ASP.NET and you might not even have known it.
Web Services
With ASP.NET Web Services Microsoft has created a number of time-saving features to make it easy to quickly write and expose web services from your application. Just like the rest of .NET, ASP.NET Web Services can be written in any .NET language. Through the use of the Web Services Extensions (free download from Microsoft), you can also add support for the new and ever-growing list of WS-* specifications to your ASP.NET Web Services.
The Tools
One of the benefits of the .NET Framework is the great tools that are available to the .NET developer. Visual Studio has long been considered one of the premier IDEs on the market and does a lot to increase developer productivity when working with the framework. There are also a large number of open source tools available for .NET, including many that mimic the tools available on other platforms. Some of these include nUnit for unit testing, nAnt for building projects, nCover for testing code coverage, nHibernate for object persistence, and much more.
Versions
Microsoft started development on the .NET Framework in the late 1990s originally under the name of Next Generation Windows Services (NGWS). By late 2000 the first beta versions of .NET 1.0 were released.
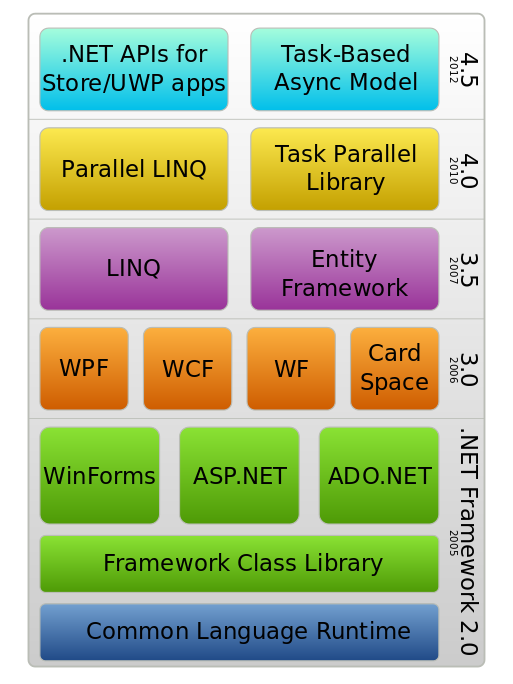
The .NET Framework stack
Version |
Version Number |
Release Date |
Visual Studio |
Default in Windows |
1.0 |
1.0.3705.0 |
2002-02-13 |
Visual Studio .NET |
N/A |
1.1 |
1.1.4322.573 |
2003-04-24 |
Visual Studio .NET 2003 |
Windows Server 2003 |
2.0 |
2.0.50727.42 |
2005-11-07 |
Visual Studio 2005 |
Windows Server 2003 R2 |
3.0 |
3.0.4506.30 |
2006-11-06 |
|
Windows Vista, Windows Server 2008 |
3.5 |
3.5.21022.8 |
2007-11-19 |
Visual Studio 2008 |
Windows 7, Windows Server 2008 R2 |
4.0 |
4.0.30319.1 |
2010-04-12 |
Visual Studio 2010 |
N/A |
4.5 |
4.5.50709.17929 |
2012-08-15 |
Visual Studio 2012 |
Windows 8, Windows Server 2012 |
A more complete listing of the releases of the .NET Framework may be found on the .NET Framework version list.
.NET Framework 1.0
This is the first release of the .NET Framework, released on 13 February 2002 and available for Windows 98, Me, NT 4.0, 2000, and XP. Mainstream support by Microsoft for this version ended 10 July 2007, and extended support ended 14 July 2009.
.NET Framework 1.1
This is the first major .NET Framework upgrade. It is available on its own as a redistributable package or in a software development kit, and was published on 3 April 2003. It is also part of the second release of Microsoft Visual Studio .NET (released as Visual Studio .NET 2003). This is the first version of the .NET Framework to be included as part of the Windows operating system, shipping with Windows Server 2003. Mainstream support for .NET Framework 1.1 ended on 14 October 2008, and extended support ends on 8 October 2013. Since .NET 1.1 is a component of Windows Server 2003, extended support for .NET 1.1 on Server 2003 will run out with that of the OS - currently 14 July 2015.
Changes in 1.1 on comparison with 1.0
- Built-in support for mobile ASP.NET controls. Previously available as an add-on for .NET Framework, now part of the framework.
- Security changes - enable Windows Forms assemblies to execute in a semi-trusted manner from the Internet, and enable Code Access Security in ASP.NET applications.
- Built-in support for ODBC and Oracle databases. Previously available as an add-on for .NET Framework 1.0, now part of the framework.
- .NET Compact Framework - a version of the .NET Framework for small devices.
- Internet Protocol version 6 (IPv6) support.
- Numerous API changes.
.NET Framework 2.0
Released with Visual Studio 2005, Microsoft SQL Server 2005, and BizTalk 2006.
- The 2.0 Redistributable Package can be downloaded for free from Microsoft, and was published on 22 January 2006.
- The 2.0 Software Development Kit (SDK) can be downloaded for free from Microsoft.
- It is included as part of Visual Studio 2005 and Microsoft SQL Server 2005.
- Version 2.0 without any Service Pack is the last version with support for Windows 98 and Windows Me. Version 2.0 with Service Pack 2 is the last version with official support for Windows 2000 although there have been some unofficial workarounds published online to use a subset of the functionality from Version 3.5 in Windows 2000. Version 2.0 with Service Pack 2 requires Windows 2000 with SP4 plus KB835732 or KB891861 update, Windows XP with SP2 or later and Windows Installer 3.1 (KB893803-v2).
- It shipped with Windows Server 2003 R2 (not installed by default).
Changes in 2.0 in comparison with 1.1
- Numerous API changes.
- A new hosting API for native applications wishing to host an instance of the .NET runtime. The new API gives a fine grain control on the behavior of the runtime with regards to multithreading, memory allocation, assembly loading and more. It was initially developed to efficiently host the runtime in Microsoft SQL Server, which implements its own scheduler and memory manager.
- Full 64-bit support for both the x64 and the IA64 hardware platforms.
- Language support for generics built directly into the .NET CLR.
- Many additional and improved ASP.NET web controls.
- New data controls with declarative data binding.
- New personalization features for ASP.NET, such as support for themes, skins and webparts.
- .NET Micro Framework - a version of the .NET Framework related to the Smart Personal Objects Technology initiative.
- Partial classes
- Anonymous methods
- Data Tables
.NET Framework 3.0
.NET Framework 3.0, formerly called WinFX, was released on 21 November 2006. It includes a new set of managed code APIs that are an integral part of Windows Vista and Windows Server 2008 operating systems. It is also available for Windows XP SP2 and Windows Server 2003 as a download. There are no major architectural changes included with this release; .NET Framework 3.0 uses the Common Language Runtime of .NET Framework 2.0. Unlike the previous major .NET releases there was no .NET Compact Framework release made as a counterpart of this version.
.NET Framework 3.0 consists of four major new components:
- Windows Presentation Foundation (WPF), formerly code-named Avalon; a new user interface subsystem and API based on XML and vector graphics, which uses 3D computer graphics hardware and Direct3D technologies.
- Windows Communication Foundation (WCF), formerly code-named Indigo; a service-oriented messaging system which allows programs to interoperate locally or remotely similar to web services.
- Windows Workflow Foundation (WF) allows for building of task automation and integrated transactions using workflows.
- Windows CardSpace, formerly code-named InfoCard; a software component which securely stores a person's digital identities and provides a unified interface for choosing the identity for a particular transaction, such as logging in to a website.
.NET Framework 3.5
Version 3.5 of the .NET Framework was released on 19 November 2007, but it is not included with Windows Server 2008. As with .NET Framework 3.0, version 3.5 uses the CLR of version 2.0. In addition, it installs .NET Framework 2.0 SP1, (installs .NET Framework 2.0 SP2 with 3.5 SP1) and .NET Framework 3.0 SP1 (installs .NET Framework 3.0 SP2 with 3.5 SP1), which adds some methods and properties to the BCL classes in version 2.0 which are required for version 3.5 features such as Language Integrated Query (LINQ). These changes do not affect applications written for version 2.0, however.
As with previous versions, a new .NET Compact Framework 3.5 was released in tandem with this update in order to provide support for additional features on Windows Mobile and Windows Embedded CE devices.
The source code of the Base Class Library in this version has been partially released (for debugging reference only) under the Microsoft Reference Source License.
Changes since version 3.0
- New language features in C# 3.0 and VB.NET 9.0 compiler
- Adds support for expression trees and lambda methods
- Extension methods
- Expression trees to represent high-level source code at runtime.
- Anonymous types with static type inference
- Language Integrated Query (LINQ) along with its various providers
- LINQ to Objects
- LINQ to XML
- LINQ to SQL
- Paging support for ADO.NET
- ADO.NET synchronization API to synchronize local caches and server side datastores
- Asynchronous network I/O API .
- Peer-to-peer networking stack, including a managed PNRP resolver.
- Managed wrappers for Windows Management Instrumentation and Active Directory APIs
- Enhanced WCF and WF runtimes, which let WCF work with POX and JSON data, and also expose WF workflows as WCF services. WCF services can be made stateful using the WF persistence model.
- Support for HTTP pipelining and syndication feeds.
- ASP.NET AJAX is included
- New
System.CodeDom
namespace.
Service Pack 1
The .NET Framework 3.5 Service Pack 1 was released on 11 August 2008. This release adds new functionality and provides performance improvements under certain conditions, especially with WPF where 20-45% improvements are expected. Two new data service components have been added, the ADO.NET Entity Framework and ADO.NET Data Services. Two new assemblies for web development, System.Web.Abstraction and System.Web.Routing, have been added; these are used in the ASP.NET MVC Framework and, reportedly, will be utilized in the future release of ASP.NET Forms applications. Service Pack 1 is included with SQL Server 2008 and Visual Studio 2008 Service Pack 1.
There is also a new variant of the .NET Framework, called the ".NET Framework Client Profile", which at 28 MB is a lot smaller than the full framework and only installs components that are the most relevant to desktop applications. However, the Client Profile amounts to this size only if using the online installer. When using the off-line installer, the download size is still 250 MB.
.NET Framework 4.0
Microsoft announced the .NET Framework 4.0 on 29 September 2008. The Public Beta was released on 20 May 2009.
In 28 July 2009, a second release of the .NET Framework 4.0 beta was made available with experimental software transactional memory support. This functionality is not available in the final version of the framework.
On 19 October 2009, Microsoft released Beta 2 of the .NET Framework 4. At the same time, Microsoft announced the expected launch date for .NET Framework 4 as the 22 March 2010. This launch date was subsequently delayed to 12 April 2010.
On 10 February 2010, a release candidate was published: Version:RC.
On 12 April 2010, the final version of .NET Framework 4.0 was launched alongside the final release of Visual Studio 2010. On 18 April 2011, version 4.0.1 was released supporting some customer-demanded fixes for Windows Workflow Foundation. Its design-time component, which requires Visual Studio 2010 SP1, adds a workflow state machine designer.
On 19 October 2011, version 4.0.2 was released supporting some new features of Microsoft SQL Server.
Some focuses of this release are:
- Parallel Extensions to improve support for parallel computing, which target multi-core or distributed systems. To this end, they plan to include technologies like PLINQ (Parallel LINQ), a parallel implementation of the LINQ engine, and Task Parallel Library, which exposes parallel constructs via method calls.
- Visual Basic and C# languages innovations such as statement lambdas, implicit line continuations, dynamic dispatch, named parameters, and optional parameters.
- Full support for IronPython, IronRuby, and F#.
- Support for a subset of the .NET Framework and ASP.NET with the "Server Core" variant of Windows Server 2008 R2.
- Support for Code Contracts.
- Inclusion of the Oslo modelling platform, along with the M programming language.
.NET Framework 4.5
.NET Framework 4.5 was released on 15 August 2012., a set of new or improved features were added into this version. The .NET Framework 4.5 is only supported on Windows Vista or later.